What is showpoint c++ ?
It basically set formatting flag for stream in str. Showpoint in c++ is used for displaying string value based on precision.
If precision is 2 then value=1 will be display as 1.0 ; so whenever in c++ program we want to display string based on precision we have to use std::showpoint if we don’t want to use precision then we can use std::noshowpoints during string printing.
std::showpoint :- It show decimal points in display. Showpoint formatting flag is set for stream display.
std::noshowpoint :- It don’t show decimal points in display. Showpoint formatting flag is unset for stream display.
Declaration of showpoint in C++
ios_base& showpoint (ios_base& str);
Parameters of showpoint c++
str is stream object whose format flag is affected.
Return Value of showpoint c++
It returns argument str.
Exceptions in showpoint c++
Basic guarantee of showpoint − if an exception is thrown in program then str is in a valid state.
Data races in showpoint c++
It modifies str. Concurrent access to the same stream object may cause data races.
Examples of showpoint C++
#include <iostream>
int main () {
double a = 12;
double b = 12345.4;
double c = 1.2345;
// precision is set to 5
std::cout.precision (5);
std::cout << std::showpoint << a << '\t' << b << '\t' << c << '\n';
std::cout << std::noshowpoint << a << '\t' << b << '\t' << c << '\n';
return 0;
}
OUTPUT:
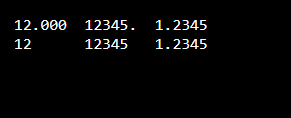
If we change precision to 6 then output will be different.
#include <iostream>
int main () {
double a = 12;
double b = 12345.4;
double c = 1.2345;
// precision is set to 6
std::cout.precision (6);
std::cout << std::showpoint << a << '\t' << b << '\t' << c << '\n';
std::cout << std::noshowpoint << a << '\t' << b << '\t' << c << '\n';
return 0;
}
OUTPUT:
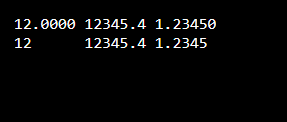
Conclusion:
Using showpoint c++ we can set flag for showing values in decimals. If value is less then precision set then other values after point, will be display as 0. If we want to unset flag then we can use noshowpoint, by this way decimal points will not be display.
For learning more c++ technical topics you can visit other articles in our website.
Keep learning and become code hunter !! 🙂
Leave a Reply