When we are calling some function but there is not matching function definition argument, then we get compilation error as No matching function for call to c++. To resolve this error, We need to pass appropriate matching argument during function call. Or need to create different overloaded function with different arguments.
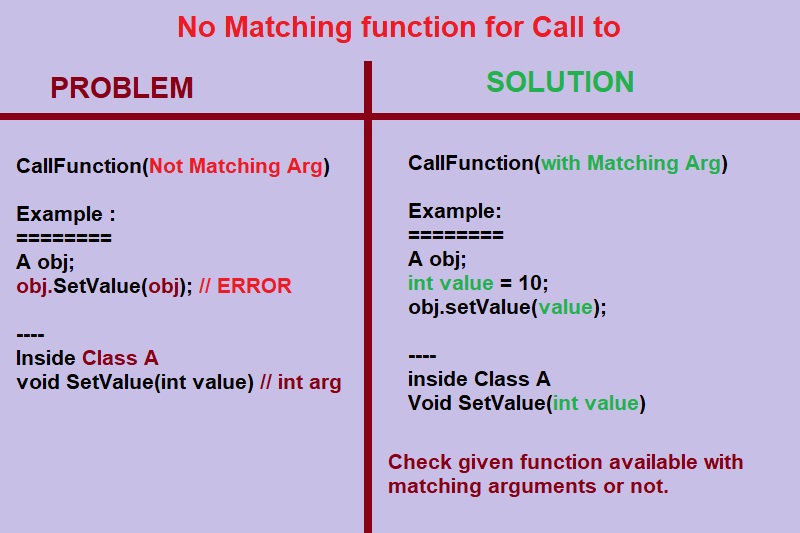
Check function calling and function definition argument data types. It must be same.
No matching function for call to c++ error
#include <iostream>
using namespace std;
class A
{
public:
void setValue(int value);
int value;
};
void A::setValue(int value)
{
value++;
}
int main(int argc, char** argv)
{
A obj;
obj.setValue(obj); // ERROR: No matching function for call to
return 0;
}
Output | error: no matching function for call to
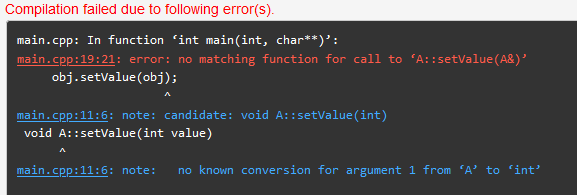
Here if you see we are passing Class object inside setValue() function calling argument. But if we check in setValue() function definition that we expect passing argument value as integer. So here function calling argument and expected arguments are not matching so we are getting error of no matching function for call to c++.
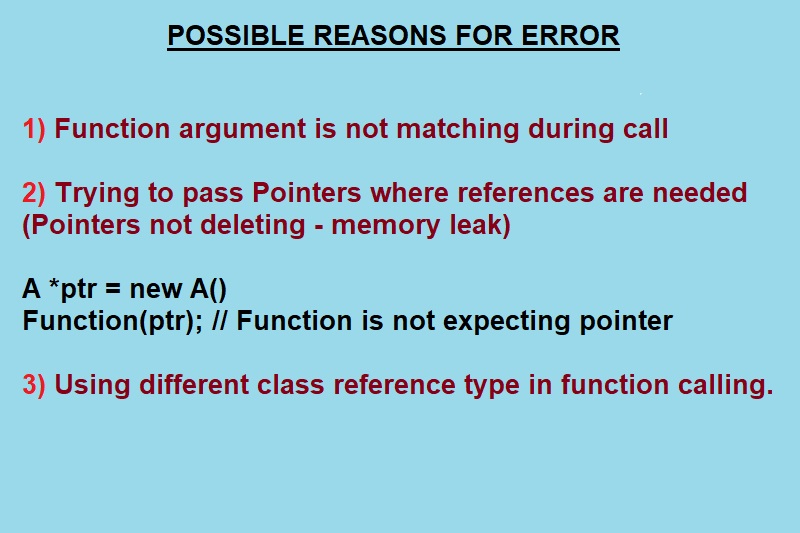
[SOLUTION] How to resolve “No matching function for call to” c++ error ?
int main(int argc, char** argv)
{
A obj;
int value=0;
obj.setValue(value);
return 0;
}
Here we just modified setValue() function argument as integer. So it will be match with function definition arguments. So no matching function for call to c++ error will be resolve.
Frequently asked queries for No Matching function for call:
1. no matching function for call to / no matching function for call
If function call and function definition arguments are not matching then you might get this error. Depend on compiler to compiler you might get different errors. Sometimes it also give that type mismatch or can not convert from one data type to another.
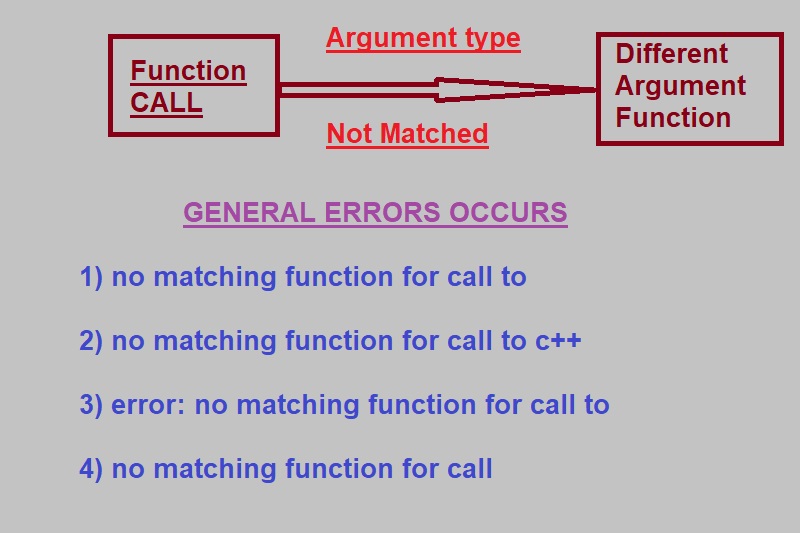
2. error: no matching function for call to
You will get error for no matching function call when generally you are passing object / pointer / reference in function call and function definition is not able to match and accept that argument.
Conclusion:
Whenever you are getting no matching function for call to c++ error then check function arguments and their data types. You must be making mistake during function calling and passing mismatch argument or you might be require to add new function with similar matching data type. After checking and adding suitable function argument change your error will be resolve. I hope this article will solve your problem, in case of any further issue or doubt you can write us in comment. Keep coding and check Mr.CodeHunter website for more c++ and programming related articles.
Leave a Reply