If you are new to c++ programming language then possibly you will come across c++ identifier is undefined errors. You might get identifier is undefined error in multiple ways, below 4 different possible errors for identifier is undefined in c++.
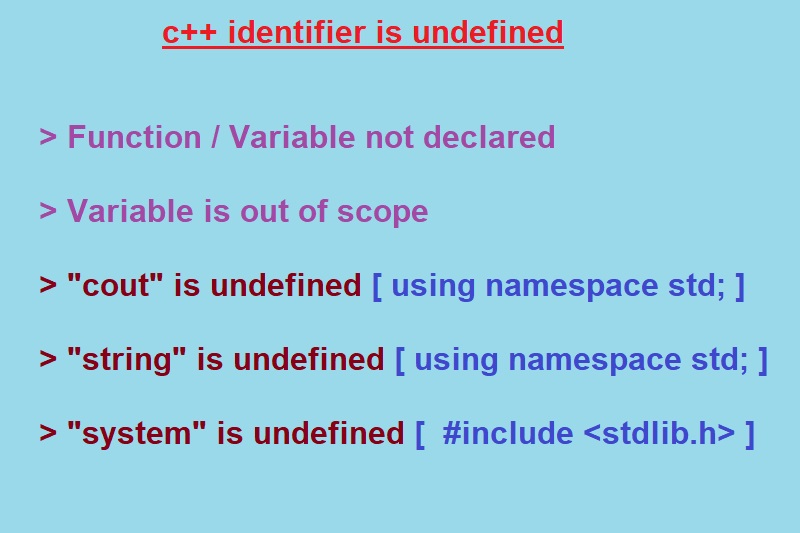
Possible different errors:
- c++ identifier is undefined
- c++ identifier cout is undefined
- c++ identifier string is undefined
- identifier system is undefined c++
1) c++ identifier is undefined
Solution-1 : identifier is undefined due to variable/function is not declared
#include <iostream>
using namespace std;
void func(int a) {
cout << a;
}
int main()
{
int Value1;
Value1 = 10;
func(Value);
// error C2065: 'Value': undeclared identifier
// Solution: func(Value1);
return 0;
}
Here we have not declared Value variable. So it is giving undeclared error. If we change it to func(Value1); then error will be resolve. Always check that particular variable is declared or not.
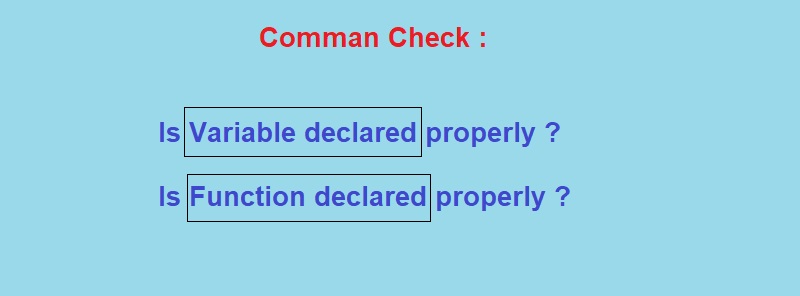
#include <iostream>
using namespace std;
int main()
{
int Value1;
Value1 = 10;
func(Value1);
// error C3861: 'func': identifier not found
return 0;
}
Here func(int value){…} is not available so it will give error for c++ identifier not found.
Solution-2 : c++ identifier is undefined due to variable is out of Scope {..}
#include <iostream>
using namespace std;
int main()
{
{
int Value1; // Variable scope will be over after {}
}
Value1 = 10;
// error C2065: 'Value1': undeclared identifier
return 0;
}
Here variable declaration scope is over once {..} is over. So outside scope if we try to access variable then it will give error for c++ identifier is undefined.
2) c++ identifier cout is undefined
#include <iostream>
int main()
{
int Value1;
Value1 = 10;
cout << Value1;
// error C2065: 'cout': undeclared identifier
return 0;
}
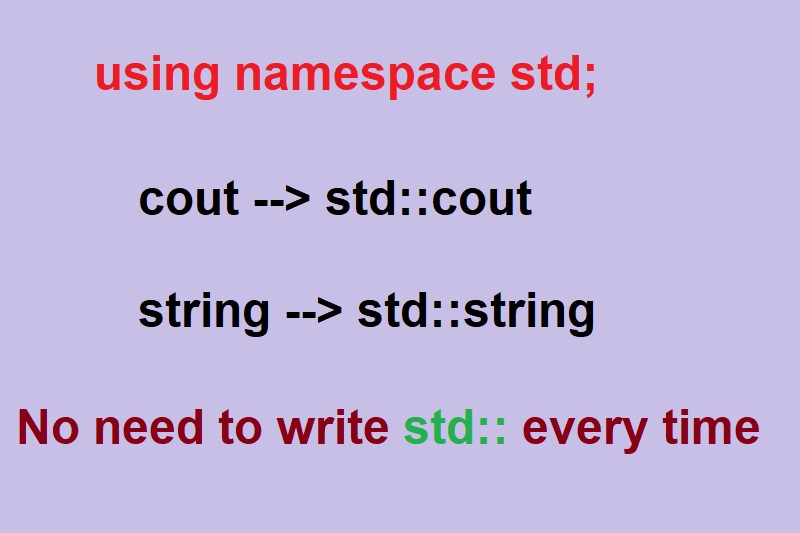
Here error is coming because cout is inside std namespace.
std::cout << Value1;
If you don’t wand to add std::cout every time then you can add using namespace std; in header section so it will apply to whole file.
#include <iostream>
using namespace std; // Need to add it
Using these both solutions you can easily remove c++ identifier cout is undefined error from your program.
3) c++ identifier string is undefined
#include <iostream>
int main()
{
string str;
// C2065: 'string': undeclared identifier
return 0;
}
string variable is also inside std namespace, so you will need to use std::string every time or you can add using namespace std; inside starting of file includes.
std::string str;
OR
using namespace std;
Using this code change you can resolve c++ identifier string is undefined from your program.
4) identifier system is undefined c++
#include <stdio.h>
int main()
{
system("pause");
// error C3861: 'system': identifier not found
// system is available inside #include <stdlib.h>
return 0;
}
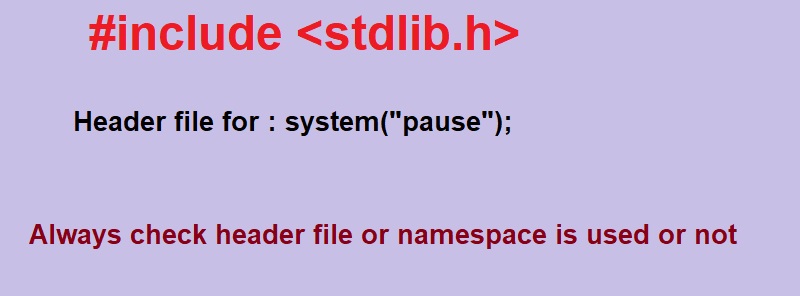
system() is define inside #include <stdlib.h> header file. If you have not included this file then you will get errors for identifier system is undefined c++.
Conclusion: c++ identifier is undefined
Whenever you are getting identifier is undefined error in c++ then you need to check 1) Variable name is declared or not 2) Variable or function is not out of scope 3) Proper #include file is added or not. Mostly due to these three reason error occurs. By checking coding you will able to identify issue.
See more:
C++ is widely used programming language, you can read more use of c++ in real world.
Leave a Reply