In c++ project when you have included header file, but inside project we are not able to access that header file then generally we get error for cannot open source file. For fixing this error check that included file is available in project include path or not.
cannot open source file visual studio C++
Solution-1 : Check your Visual Studio Project settings under C++, Check Include directories and make sure Your_filename.h is pointing to correct path. After adding proper include directories it will resolve cannot open source file visual studio error.

You can right click to header file and open from visual studio. If file is pointing properly it will open.
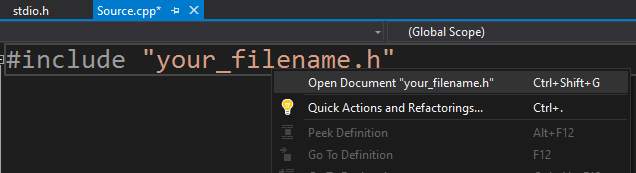
If your include file is not properly pointing or you have not added inside your included directory then you will not able to open from visual studio.
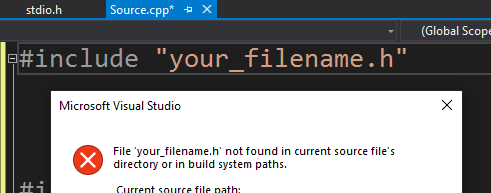
Solution-2 : Another solution, If your header file is at different folder/location then you can include file path directly in header file. Here you can use relative path or you can provide full path of header file.
Include based on your project folder structure and requirement.
#include "../FolderName/filename.h"
OR
#include "./FolderName/filename.h"
OR
#include "FullPath/filename.h"
c++ cannot open source file iostream
Solution-1: iostream file opening error normally comes when we are missing $(IncludePath) inside Properties->VC++ Directories->Include Directories. And you have by mistake removed Include path.
Solution-2: Other possible reason is that during installing Visual studio you did not selected c++ packages.
Solution-3: You can check by including stdafx.h in starting (only for Visual studio projects)
#include "stdafx.h"
#include <iostream>
using namespace std;
Solution-4: Sometimes these type of error comes when multiple Visual Studio versions are install in single PC. You can check Projects and Solutions –> VC++ Directories, are properly added for your project and pointing to correct version location.
For Visual Studio Code:
c++ cannot open source file iostream in visual studio code can also occurs when proper paths are not added inside visual studio code c_cpp_properties.json
file.
Mode details:
https://code.visualstudio.com/docs/cpp/config-msvc#_prerequisites
cannot open source file “string”
Try below steps to resolve error.
1) Open VC++ Directories option inside Configuration Properties of visual studio
2) Her all directories value (e.g. Executable directories) will be available, just you need to select drop-down and click edit
3) Remove selection of Inherit from parent
4) Now just click OK and you will see no changes inside Edit box.
5) You need to repeat this thing for all directories, At the end executable directories will be set to $(ExecutablePath) and Include directories will be set to $(IncludePath), similar way to all directories
6)Now click apply and then OK
Conclusion for cannot open source file c++
We have provided solutions for multiple errors for can not open source file c++, If you try given solution hopefully you will able to resolve error. But if still you are getting error you can add your comment and code. We will try to resolve and find solution for you. Happy coding ๐
Hi there from ‘down under’. I’ve just installed VS2022.
However, I am getting error E1696: ‘cannot open source file “string.h” , ‘stdlib.h’ etc
FYI I have been using Vs2019 and C# without probs.
I also have visual studio code installed.
Do I have to uninstall it/them?
In my Wondows10 Environment Variables I have ‘VISUAL_STUDIO_REPOS’ defined where my projects are stored. Is there something else needing adding/deleting?
Ta
Hello Mark,
Ok so what i understood, it is working proper with VS2019 but giving issue with VS2022. So then you need to make sure that You have installed Visual studio proper and Also check Windows SDK update require or not for new Version. Check this article points it might be helpful in your case: https://mrcodehunter.com/cannot-open-source-file-errno-h/
Please check if latest Windows 10 SDK is required for same or not.
No ideally not require to uninstall visual studio code. As you are running code in Visual Studio 2022.
Also make sure you install Visual C++ during installation.
Also once check given MSDN URL: https://social.msdn.microsoft.com/Forums/en-US/6ea597fe-fda5-464f-bcb7-a9b88bc7bf83/please-help-how-to-fix-error-e1696-file-source-cannot-be-opened-quotiostreamquot?forum=visualstudiogeneral
i have just downloaded the open cv and trying to use it’s header files ,it showing me error in the top 2 header files , i tried the way you mentioned above but it sill not working , please sir help me if you can . i included the header file folder in additional include directories but i also have project include directories folder there
#include
#include
#include
#include
using namespace cv;
using namespace std;
int main()
{
string path = “Resources/test.png”;
Mat img = imread(path);
imshow(“Image”, img);
waitKey(0);
return 0;
}
Can you give more details for error ? Try everything and try to find that file is available in which location. Might be it will be in OpenCV folder. Generally by setting Include directory path it should be resolved. Did you check anything is missing for OpenCV configuration setup ?
#include
#include
#include
#include
#include “GenerateArray.h”-
#include “ArrayOutput.h”- this 3 is doesnt work
#include “SortArray.h”-
int main()
{
int size = 40;
int* arr = new int[size];
int s = 0;
do
{
printf(“1) Generate an array\n”);
printf(“2) Display the array on the screen\n”);
printf(“3) Sort array\n”);
printf(“4) Display the sorted array\n”);
printf(“5) Press zero to exit\n\n”);
scanf_s(“%d”, &s);
switch (s)
{
case 1:
GenerateArray(size, arr);
system(“CLS”);
break;
case 2:
system(“CLS”);
ArrayOutput(size, arr);
printf(“\n”);
break;
case 3:
SortArray(size, arr);
system(“CLS”);
break;
case 4:
system(“CLS”);
ArrayOutput(size, arr);
printf(“\n”);
break;
}
} while (s > 0);
}
Hello Stepan,
Can you check:
1) ArrayOutput.h file is available in current directory? check path of file
2) Try to right click and open file, If it does not found you will get popup in visual studio that fine not found
3) Try to give full path of file like D:/Data/…etc/ArrayOutput.h
#include
#include // this line is error, cannot open source file studio.h
#include
void draw() {
glClear(GL_COLOR_BUFFER_BIT);
glLoadIdentity();
glOrtho(0, 4, 0, 4, -1, 1);
glBegin(GL_POLYGON);
glVertex2i(1, 1);
glVertex2i(3, 1);
glVertex2i(3, 3);
glVertex2i(1, 3);
glEnd();
glFlush();
}
int main(int argc, char** argv) {
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_SINGLE | GLUT_RGBA);
glutCreateWindow(“whitesquare”);
glutDisplayFunc(draw);
glutMainLoop();
}
studio.h is external library ? If yes then you need to add in your Project property for additional dependency library.
#include
using namespace std;
int main()
{
int rows = 0;
int Maxdigit = 9;
cout <> rows;
while (rows % 2 == 0 || rows < 5)
{
cout << "Invalid Input. The entered number should be odd and greater than 5";
cout << endl;
cout <> rows;
}
if (rows != 7)
{
for (int i = 1; i <= rows; i = i + 2)
{
cout << " ";
}
cout << "1";
for (int i = 1; i <= rows; i = i + 2)
{
cout << " ";
}
cout << endl;
for (int i = 3; i <= rows; i = i + 2)
{
cout << " ";
}
for (int j = 3; j <= Maxdigit – 2; j = j + 2)
{
cout << j;
if (j == 7)
{
cout << endl;
cout <= 1; i = i – 2)
{
cout << i;
if (i == 1)
{
cout << endl;
cout << " ";
for (int j = i + 2; j <= 9; j = j + 2)
{
cout << j;
int count = count + 1;
if (count == rows)
{
for (int i = j + 2; j <= 9; j++)
{
for (int k = j; k <= j; k = k + 2)
{
cout << k;
}
cout << endl;
}
}
if (j == 7)
{
cout << endl;
cout << " ";
}
}
}
}
cout << " " << endl;
}
}
for (int k = 1; k <= rows; k = k + 2)
{
cout << " ";
}
}
if (rows == 7)
{
for (int i = 1; i <= rows; i = i + 2)
{
cout << " ";
}
cout << "1";
for (int i = 1; i <= rows; i = i + 2)
{
cout << " ";
}
cout << endl;
for (int i = 3; i <= rows; i = i + 2)
{
cout << " ";
}
for (int j = 3; j <= Maxdigit – 2; j = j + 2)
{
cout << j;
if (j == 7)
{
cout << endl;
cout <= 1; i = i – 2)
{
cout << i;
if (i == 1)
{
cout << endl;
cout << " ";
for (int j = i + 2; j <= 9; j = j + 2)
{
cout << j;
}
for (int j = 1; j <= 5; j = j + 2)
{
cout << j;
}
cout << endl;
cout << " ";
for (int i = Maxdigit – 2; i <= 9; i = i + 2)
{
cout <= 3; j = j – 2)
{
cout << j;
}
cout << endl;
cout << " ";
for (int i = 5; i <= 9; i = i + 2)
{
cout << i;
}
cout << endl;
cout << " 1";
}
}
}
}
}
system("pause");
return 0;
}
Can you tell me if you are getting any issue ? did not understood your comment. You just posted code.