In c++ logic when we require square of a number then there are multiple solutions are available for it.
- You can use Power function of c++ pow(Base,Power)
- Simple multiplication of number two time
- If we want to make square without multiplication then we can achieve it using for loop or while loop.
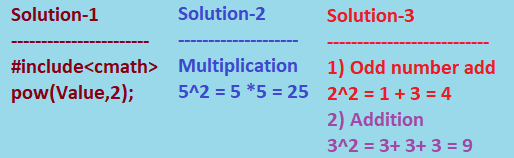
How to square a number in c++ ?
#include<iostream>
#include<cmath>
using namespace std;
int main(){
float B = 10.0;
float P = 2.0;
float Square_of_Number = pow(B,P);
//B ^P = 10^2 = 100
cout<<"Square of Number = "<<Square_of_Number<<endl;
}
Here we can square number using Power function. You will need to include #include<cmath> for it. Inside function we need to pass Base value which we want to square and Power value (for square it will be 2). So example will be Pow(Power,Base). You can consider it as c++ square operator.
square function c++
#include<iostream>
using namespace std;
float Square(float value){
// Multiply value two times
return value*value;
}
int main(){
cout<<"Square of Number = "<<Square(10)<<endl;
}
We can create separate function for getting square of number. Here we just have to do multiplication of number two time.
Find square of a number without using multiplication or division operators
If there is requirement to create square of number without using multiplication/division then we have to create different logic using for loop or while loop.
- Using for loop, make addition of number
- Using while loop, we have to add Odd number so it will generate square at the end.
c++ program to find square of a number using for loop
#include<iostream>
using namespace std;
float Square(float value){
float ans=0;
// 10+10...10 time addition
for(int i=0;i<value;i++){
ans= ans + value;
}
return ans;
}
int main(){
cout<<"Square of Number = "<<Square(10)<<endl;
}
Here we are adding 10+10…. up to 10 times. So after addition it will create square of 10 which is 100.
c++ program to find a square of a number using while loop
#include <iostream>
using namespace std;
int Square(int Number)
{
int OddNumber = 1;
int SquareValue = 0;
// If Value is negative : Convert to Positive
Number = abs(Number);
// add odd numbers num times to result
// 3^2 = (1 + 3 + 5) = 9
while (Number--)
{
SquareValue = SquareValue + OddNumber;
OddNumber = OddNumber + 2; // Adding 2 to make it odd
}
return SquareValue;
}
int main()
{
cout<<"Square of Number = "<<Square(3)<<endl;
return 0;
}
Here we are adding odd number using while loop and final result will create square of a number.
Leave a Reply