In c++ development system(“pause”) is used to execute pause command of Operating system inside program. So it will ask user to press any key to continue. If we don’t want to use system pause c++ then we can use cin.get() which also wait for user to press any key.
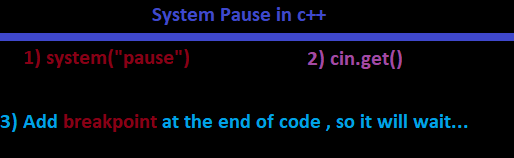
#include<iostream>
using namespace std;
int main()
{
cout << "system pause 1" << endl;
system("pause"); // press any key to continue...
cout << "system pause 2" << endl;
cin.get(); // blank screen wait for any key press
}
Output:
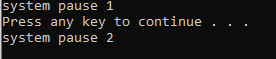
int system(const char *command);
system() is called operating system command in c/c++. If you are getting error to use it you will require to include header file stdlib.h or cstdlib.
Disadvantages of using system(“pause”) in c/c++
- It use resource heavily function call. So it is expensive if our application is performance critical.
- It is not portable. If we use system(“pause”) then it will work for windows operating system only, it pause command will not work for Linux or Mac OS.
Alternative for system pause c++
cin.get();
- You can use cin.get() for waiting to user to press any key.
- Try to add breakpoints at end of program. So during debugging it will wait at the end of program so you can see output in your command line output.
CONCLUSION:
Many times in c++ command line output we require wait for looking output of program. For adding some pause in program we can use three different ways.
- system pause c++ used by system(“pause”) command.
- If we want any other solution and not want to use system(“pause”) then we can use cin.get(). It will also wait for user to press any key. But it will not display message in command line similar to system pause command.
- If we are debugging our c++ program and just want some pause to take a look our command line program output then we can just add breakpoint at the end of program. So we out command line output will not be closed directly and it will wait at the end. So we can easily see output of our program and can proceed further using debugging easily.
Leave a Reply