In c++ class if we want to call any function then we can call it by creating object. But if function is static, then we can call by ClassName::FunctionName. If non-static function we are call by class::function then it will give error of c++ a nonstatic member reference must be relative to a specific object.
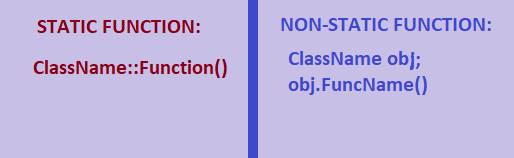
#include <iostream>
using namespace std;
class A {
public:
static void Print();
void Show();
};
void A::Print() {
cout << "Print" << endl;
}
void A::Show() {
cout << "Show" << endl;
}
int main() {
// Static call by ClassName::Func()
A::Print();
// error C2352: 'A::Show': illegal call of non-static member function
A::Show();
// Non Static call by Obj
A obj;
obj.Show();
return 0;
}
How to Solve this Error ?
- You can create Show() function as static function and then call it by class name and scope resolution(::)
- You can create class object and then call function by objectName.Function()
Why Static function require to call using scope resolution(::) ?
For static function we need to call function by ClassName::Function, because static function is not attached to particular object. So they does not contain this pointer (*this). Static member functions can directly access to any of other static members variables or functions , but it can not access other non-static members. This is because all non-static functions are attached with object of class so we will require to call it using object.Func.
Conclusion:
Whenever we are getting c++ a nonstatic member reference must be relative to a specific object error in our program we need to use class object to call it or we can make particular function as static, everything is depend on requirement of our code and project.
Leave a Reply