When any function or statement is not in scope or we have used wrong syntax then possibly you will get error for c++ expected a declaration in your code.
Main reasons for errors are:
- Incorrect use/declaration inside namespace
- Statements are added out of scope
- Required statement need to add inside main/function
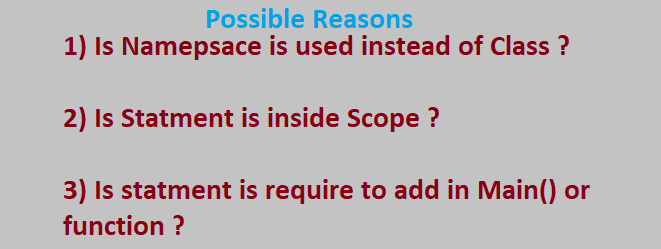
Solution-1 | Expected a declaration – When used namespace instead of class.
When developer is mostly worked in java/C# then possibly then make mistake in c++ code for namespace. If we check here given syntax is not correct for name space. For c++ we use public: for any functions.
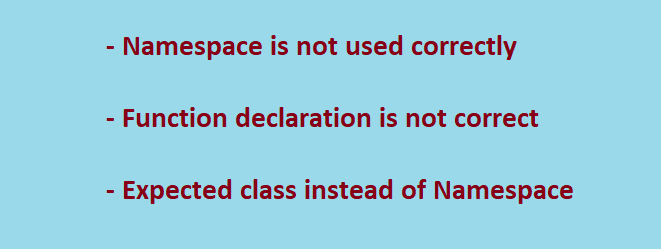
namespace A {
// Expected declaration error
public static void Print();
};
c++ expected a declaration error will come here. Here if we want to resolve error then instead of namespace we can use class. Inside Class A we can add public access specifier using colon(:) then we have to write methods. Here public static void print() is not valid in c++ so it will give error for your c++ code.
// Error resolved by using class and making function as public:
class A {
public:
static void Print();
};
Solution-2 | Expected a declaration c++ – When statement is not inside function and added out of scope
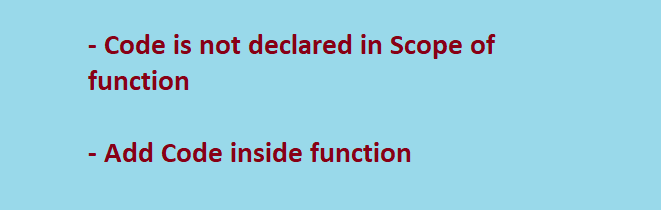
Here at place of if condition it is giving us error that declaration is expected. If you see give code then you will realize that condition is not in any scope and if require inside some function.
#include <iostream>
using namespace std;
int main() {
return 0;
}
// Expected a declaration error
if (true) {
cout << "Hello";
}
Here we have used if statement out side main() and also it is not inside any function. So it is out of scope. We will either require to add given code inside any of function or we can add inside main function then only expected a declaration error will be resolved.
We are successfully able to resolve this error by adding our if condition logic inside void func() , now you can use this function call to execute your logic.
#include <iostream>
using namespace std;
int main() {
func();
return 0;
}
// Resolve by adding function
void func() {
if (true) {
cout << "Hello";
}
}
Solution-3 | c++ Expected a declaration – When return statement is out side main
Here we have added return 0 outside main function. So it is giving us error for expected a declaration. Because return statement is require inside of any function. We can not write direct statement which is out of scope.
#include <iostream>
using namespace std;
int main() {
// Return is added outside main
}
// expected a declarion - syntax error return
return 0;
For solving this issue you can add return inside your main function. If similar errors you are getting for other class/function then you can first check given statement is inside scope or it require to add in any function.
int main() {
// Resolving it
return 0;
}
CONCLUSION:
Whenever you get error for c++ expected a declaration then you can check that your error indicated code is inside function scope ? or you have used namespace improperly. Mostly by checking it you will find reason for error and can resolve easily by adding code inside scope.
SEE MORE:
Leave a Reply