C++ map is part of Standard Template Library (STL). It is type of Associative container. Map in c++ is used to store unique key and it’s value in data structure. But if you want to store non-unique key value then you can use Multi Map in c++. Let us first understand in detail what is map in c++ by examples.
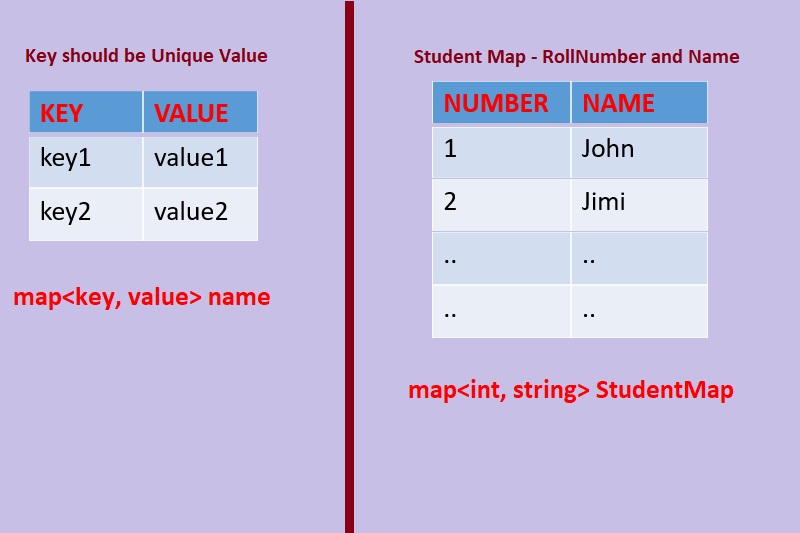
In given image you can see that c++ map can store key and value pair. Just assume you have dynamic array which can store key and value where key and value can be with different data types. Here in Student Map example we are storing student roll Number as KEY and Name of student as Value. So for whole class we can store student number and name easily. It is sure that you can not insert similar Number again in map data structure.
Syntax of c++ map
// Require header file inclusion
#include <map>
// Syntax of Map
map<key, value> ObjectOfMap;
// Examples
map<int, string> obj1; // Integer Key and String Value
map<int, int> obj2; // Key and value both Integer
For using map in c++ code you will require to add #include <map> inside your header. Map is internal implemented with Template in c++, so you will see “< >” open and closed angle in map syntax. So you can pass any dynamically data type inside it easily and it will work for all data typed generically.
Different functions supported by c++ map
Map c++ support different types of functions which can be performed data stored in map.
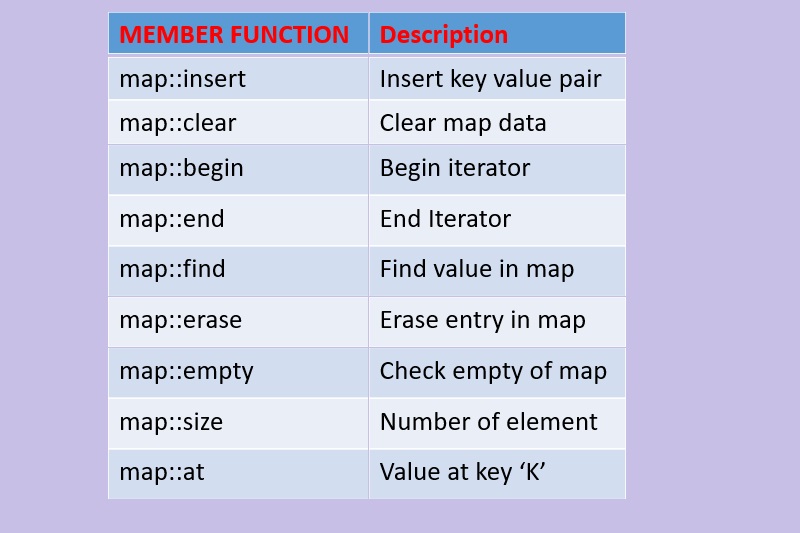
These are just examples of few map functions but there are many more functions available for c++ map.
map::insert :- It is use to insert key value pair data inside map.
map::clear :- We can clear map data using this function.
map::begin :- It return iterator of map beginning. Use to iterate map.
map::end :- It return iterator of map ending. Use to iterate map.
map::find :- Use to find key value pair from map.
map::erase :- Use to erase data from map.
map::empty :- Check map is empty or not.
map::size :- Get elements available in map.
map::at :- Get value at particular key.
How to insert in c++ map ?
Using map::insert function and using std::make_pair or pair we can insert data in map.
#include<iostream>
#include<map>
using namespace std;
int main()
{
// Create Map object
map<int, string> ObjMap;
//Insert key-value data inside map
ObjMap.insert(std::make_pair(1, "John"));
cout << "Value: " << ObjMap[1].c_str();
}
How to insert structure data in c++ Map ?
We can insert structure of data inside value of map. So key will be unique and it’s value contain structure of data, which can be bunch of multiple data.
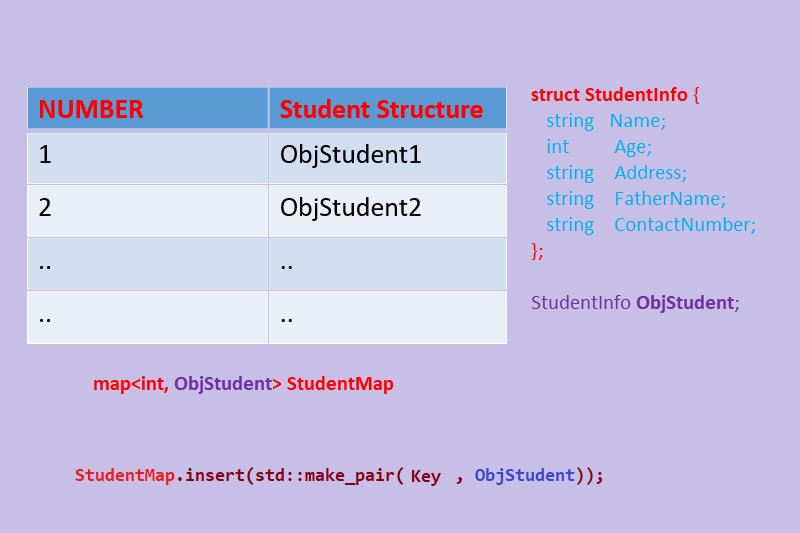
Here we are storing full student information inside map using storing StudentInfo structure object.
#include<iostream>
#include<map>
using namespace std;
// Student information structure
struct StudentInfo {
string Name;
int Age;
string Address;
string FatherName;
string ContactNumber;
};
int main()
{
// Object of structure
StudentInfo ObjStudent;
// Adding data inside structure
ObjStudent.Name = "John";
ObjStudent.Age = 25;
ObjStudent.FatherName = "Miller";
ObjStudent.Address= "N-404, Sigma city";
ObjStudent.ContactNumber = "5252552";
// Creating Map
map<int, StudentInfo> StudentMap;
// Inserting data into map
StudentMap.insert(std::make_pair(1, ObjStudent));
return 0;
}
Map iterator in c++
If we want to make loop of map from beginning to end then we have to use iterator to iterate map from begin() to end().
#include<iostream>
#include<map>
using namespace std;
int main()
{
// Create Map object
map<int, string> ObjMap;
//Insert key-value data inside map
ObjMap.insert(std::make_pair(1, "John"));
ObjMap.insert(std::make_pair(2, "Jimi"));
// Create Map iterator
map<int, string>::iterator itr;
// Loop iterator for map
for (itr = ObjMap.begin(); itr != ObjMap.end(); ++itr)
{
printf("%d : '%s'\n", (*itr).first, (*itr).second.c_str());
}
// Output
// 1 : John
// 2 : Jimi
return 0;
}
Using c++11/c++14 you can iterate map without using iterator. You can use auto keyword which will automatically understand type of returning value. It will understand that it is iterator and then it will work accordingly.
// C++11 / C++14
for (const auto& kv : ObjMap)
{
printf("%d : '%s'\n", kv.first, kv.second.c_str());
}
Find in map c++ / c++ map check if key exist
We can find value in map using map::find(key) function. We need to pass key inside find function and then check that it is not map::end(). If it reach to end and still not found value then it is clear then key is not found.
// Create Map object
map<int, string> ObjMap;
//Insert key-value data inside map
ObjMap.insert(std::make_pair(1, "John"));
ObjMap.insert(std::make_pair(2, "Jimi"));
// Create Map iterator
map<int, string>::iterator itr;
// Assign find iterator value
itr = ObjMap.find(2);
// Check if iterator is Ended then not found
if (itr != ObjMap.end())
{
cout << "Found";
}
else {
cout << "Not Found";
}
c++ map get value by key
We can get value by map:: find function. It return iterator and from iterator first and second value we can find key and value information easily.
// Create Map object
map<int, string> ObjMap;
//Insert key-value data inside map
ObjMap.insert(std::make_pair(1, "John"));
ObjMap.insert(std::make_pair(2, "Jimi"));
// Create Map iterator
map<int, string>::iterator itr;
// assign find iterator value
itr = ObjMap.find(2);
// If found
if (itr != ObjMap.end())
{
printf("%d : '%s'\n", itr->first, itr->second.c_str());
//Output:
// 2 : Jimi
}
Conclusion of c++ map
- c++ map is part of STL (Standard Template Library).
- c++ map is used to store unique key-value pair.
- Require to include #include <map> header file
- It contain many functions like insert, find, size ..etc
Leave a Reply