In c++ whenever you are calling any function of class then there might be possibility that you came across error for c++ expression must have class type. For resolving expression must have class type error you need to check that if you have class pointer then you need to use -> and if you have class object then you need to use dot(.).
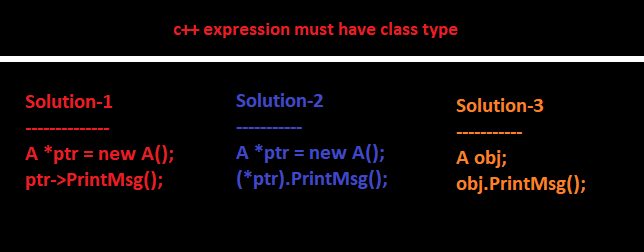
#include <iostream>
using namespace std;
class A{
public:
void PrintMsg(){
cout<<"Hello MrCodeHunter.com";
}
};
int main()
{
A *ptr = new A();
ptr.PrintMsg(); // Error is here
return 0;
}
Output:

Here we are getting error for Expression must have class type, the reason is we are using dot(.) operator for calling function of class. But we are using pointer of class. We need to make sure when we are using pointer of class then we need to use -> operator to call function.
Solution for expression must have class type error c++
int main()
{
A *ptr = new A();
ptr->PrintMsg();
// Need to use -> for calling function
return 0;
}
Here we are using class pointer so instead of dot(.) operator we need to use -> operator.
If you are using pointer and then want to call using dot(.) then also it is possible.
A *ptr = new A();
(*ptr).PrintMsg();
Here you are using (*ptr) for calling function using dot(.) which is valid scenario and your error for expression must have class type will be removed by this change also.
int main()
{
A obj;
obj.PrintMsg();
//Create object of class and call function using dot(.)
return 0;
}
If we are using class object then we can directly call class function using dot(.).
For more c++ related solutions are articles please check our website. I hope given detail will be useful for you to resolve error for expression must have class type if still you are getting error please add you comment, we will try to help you. Happy Coding ๐
Leave a Reply