Normally these type of c++ error occurs in conditional statments. For any conditional expression if we are using assignment operator(=) in-stand of comparison (==) then mostly c++ expression must be a modifiable lvalue error occurs.
1) c++ expression must be a modifiable lvalue (Scenario-1)
#include <iostream>
using namespace std;
int main()
{
int A = 0;
int B = 1;
int C = 1;
// It is same as if ( (A + B) = C )
if ( A + B = C ) // ERROR
{
std::cout << " Comparison match" << std::endl;
}
return 0;
}
Output:
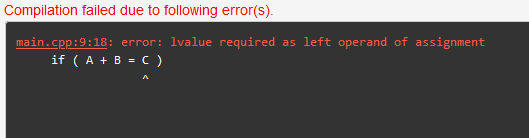
Here we are assigning C variable to expression (A+B) so compiler is giving error of lvalue required as left operand of assignment. if we want to resolve this error then we have to use comparison (==) operator. Also always make sure to add brackets “(” and “)” for easy understanding and also to avoid any operator precedence errors.
SOLUTION :
if ( (A + B) == C ) // SOLUTION using (==)
{
std::cout << " Comparison match" << std::endl;
}
By modifying this code we can easily resolve lvalue required as left operand of assignment error from condition.
2) c++ expression must be a modifiable lvalue (Scenario-2)
#include <iostream>
using namespace std;
int main()
{
int A = 0;
int B = 1;
int C = 1;
// Same as if ( (A == 0 && B) = C )
if ( A == 0 && B = C ) // ERROR
{
std::cout << " Comparision match" << std::endl;
}
return 0;
}
Output:
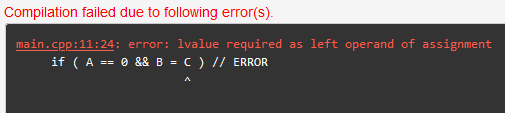
SOLUTION :
if ( (A == 0) && (B == C) ) // SOLUTION using (==)
{
std::cout << " Comparision match" << std::endl;
}
Here assignment(=) operator has lower precedence then &&. So if we change assignment operator to comparison (==) operator then it has higher precedence so (A==0) && (B==C) condition will work proper and expression must be a modifiable lvalue error will be resolved.
CONCLUSION:
Whenever your c++ compiler get error for expression must be a modifiable lvalue, you always need to check below points
- Check Assignment (=) operator is used in stand of comparison operator (==)
- Check Operator precedence for expression is correctly applied
- Try to use bracket for each expression and comparisons for avoiding mistakes
SEE MORE:
https://mrcodehunter.com/what-is-cpp-used-for-real-world-applications/
#include
int main() {
int x;
std::cin >> x;
std::cout << "pick a number";
int a;
x * 2 = a;
std::cout << "now its " << a;
}
Hello Jason,
Can you give more detail. Are you facing any issue or error ?