Fibonacci Sequence c++ is a number sequence which created by sum of previous two numbers. First two number of series are 0 and 1. And then using these two number Fibonacci series is create like 0, 1, (0+1)=1, (1+1)=2, (2+1)=3, (2+3)=5 …etc
Displaying Fibonacci Series in C++ ( without recursion)
#include<iostream>
using namespace std;
void fib(int N) {
int first = 0, second = 1, next;
for (int Index = 0; Index < N; Index++)
{
if (Index <= 1)
next = Index;
else
{
next = first + second;
first = second;
second = next;
}
cout << next << ' ';
}
}
int main()
{
int Number, Index = 0;
cout << "Enter number for fibonacci terms : ";
cin >> Number;
cout << "\nFibonacci Sequence is :\n";
fib(Number);
return 0;
}
Output:
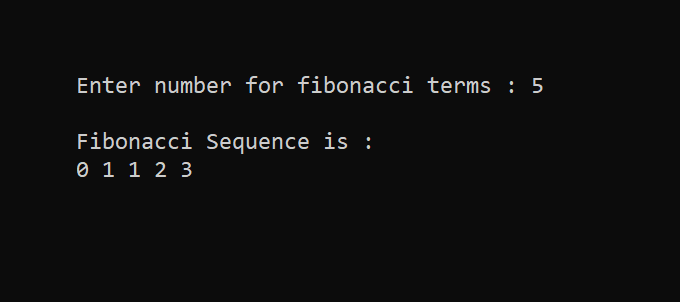
From given output we can see then sequence is created using additions of previous two digits. And it will continue by this logic then Fibonacci series will be created.
Fibonacci Series c++ recursion
We can also create Fibonacci sequence c++ using recursion operation. Recursion is calling same function with different argument withing that function. It will call in loop and then recursively calculate value.
#include<iostream>
using namespace std;
int fib(int n)
{
if ((n == 1) || (n == 0))
{
return n;
}
else
{
return(fib(n - 1) + fib(n - 2));
}
}
int main()
{
int Number, Index = 0;
cout << "Enter number for fibonacci terms : ";
cin >> Number;
cout << "\nFibonacci Sequence is :\n";
while (Index < Number)
{
cout << " " << fib(Index);
Index++;
}
return 0;
}
Given program will also create same output as regular program. Because it will just calculate sequence as recursively but output will be remain same.
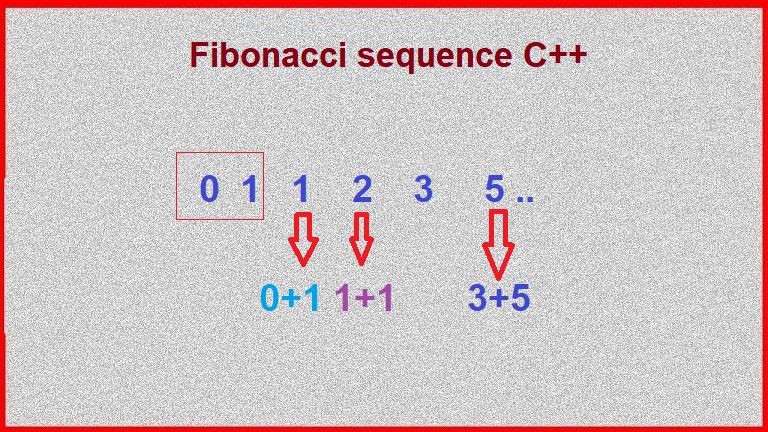
Conclusion of Fibonacci series c++
In Fibonacci sequence first two digits will be 0 and 1. Then onward 3rd digit sequence will be created using addition of previous two numbers. So in our case if we enter terms as 5 then total 5 number of sequence will be display which are 0,1,1,2,3 and 5. Hope you understand both recursive and non recursive way of generating Fibonacci series in c++. If you have any other questions or suggestion please provide your comment here. Also you can contact us if you want any other articles or suggestions in mind to create different articles.
Leave a Reply