Many time during running c++ program you get comparison errors. Sometimes it will be iso c++ forbids comparison between pointer and integer or sometimes you will get iso c++ forbids comparison between pointer and integer [-fpermissive]. Here we will see when these type of c++ compiler error occurs and how to resolve these iso c++ forbids comparison between pointer and integer errors from c++ program.
1. iso c++ forbids comparison between pointer and integer
#include <iostream>
using namespace std;
int main() {
char str[2];
cout << "Enter ab string";
cin >> str;
if (str == 'ab')
{
cout << "correct";
} else {
cout << "In correct";
}
return 0;
}
Output:
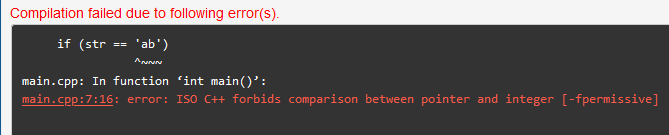
Here during string comparison you are getting compilation errors. “error: ISO C++ forbids comparison between pointer and integer [-fpermissive]”
SOLUTION FOR ERROR:
if(str==’ab’), here str is const char* type. Which is array of character.
Here ‘ab’ is consider as integer value. ideally it should be as constant string value.
Because of single quote it is considered as integer value.
So for comparison you need to use double quote “ab”. And declare it as string variable.
Now you can compare using strcmp(str, “ab”) or using str == “ab”.
#include <iostream>
using namespace std;
int main() {
string str;
cout << "Enter ab string: ";
cin >> str;
if (str == "ab")
{
cout << "correct";
} else {
cout << "In correct";
}
return 0;
}
Output:
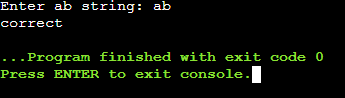
2. error: ISO C++ forbids comparison between pointer and integer [-fpermissive]
#include <iostream>
using namespace std;
int func(int i, int j) {
int val = 1;
if (val > func) {
return (val+1);
}
return val;
}
int main() {
int val = func(3,6);
return 0;
}
Output:

SOLUTION FOR ERROR:
If you see if condition if(val > func), then here we have by mistake given function name which has different datatype then integer value.
So our comparison is wrong. Whenever any comparison is wrong you will get this error. Ideally here we need to compare with some integer value.
int val = 1;
int IntValue = 0;
if (val > IntValue) {
return (val+1);
}
return val;
Here we have removed function name and comparing it with Integer value. Now that error will be resolved.
CONCLUSION:
Whenever you are getting any error similar to ISO C++ forbids comparison between pointer and integer [-fpermissive], Then check that comparison condition. You will get to know that comparison condition is mismatch with two different data type of variables. For resolving c++ forbids comparison error you just need to compare similar kind of variables in conditions. I hope given two examples will be helpful for your to find out your code issue. If you like this article then please check other c++ related information in given website. Happy Coding !! ๐
Leave a Reply