There are different ways to calculate string length c++. Here we will explain all possible solutions to calculate string length in c++.
String value end with null or \0 character. So it can also useful for c++ string length calculation.
1. string length c++ using string::size() method
#include <iostream>
#include <string.h>
using namespace std;
int main()
{
// String obj
string str1 = "string length c++";
string str2 = "12345";
// 1. Using size() method
cout << "string length c++ = "<< str1.size() << endl;
cout << "12345 = "<< str2.size() << endl;
return 0;
}
Output:
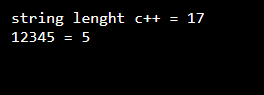
string:size c++ function
size_t size() const;
Return Value:
It returns the length of the string, which is in terms of bytes.
2. string length c++ using string::length() method
#include <iostream>
#include <string.h>
using namespace std;
int main()
{
// String obj
string str1 = "string length c++";
string str2 = "12345";
// 2. Using length() method
cout << "string length c++ = "<< str1.length() << endl;
cout << "12345 = "<< str2.length() << endl;
return 0;
}
Output:
string length c++ = 17 12345 = 5 string:length c++ function size_t size() const; Return Value: It returns the length of the string, which is in terms of bytes.
3. string length c++ using strlen() method
// String obj
string str1 = "string length c++";
string str2 = "12345";
// 3. Using strlen(string) method
cout << "string length c++ = "<< strlen(str1.c_str()) << endl;
cout << "12345 = "<< strlen(str2.c_str()) << endl;
strlen c++
Returns the length of the C string str.
size_t strlen ( const char * str );
4. string length c++ without using any string function (using while loop)
#include <iostream>
#include <string.h>
using namespace std;
int main()
{
// String obj
string str = "string length c++";
// 4. Using While Loop untill string is not null
int Index = 0;
while (str[Index] != NULL){
Index++;
}
cout <<"string length c++ = "<< Index << endl;
return 0;
}
Output:
string length c++ = 17
If we want to calculate length of string without using any of string function, then we can use while loop to iterate string until it is not null, and then print the final increased index.
5. string length c++ without using any string function (using for loop)
#include <iostream>
#include <string.h>
using namespace std;
int main()
{
// String obj
string str = "string length c++";
// 5. Using For Loop Index increase upto string is not null
int Index = 0;
for (Index = 0; str[Index] != NULL; Index++);
// After for loop over index will be lenght of string
cout <<"string length c++ = "<< Index << endl;
return 0;
}
Output:
string length c++ = 17
FAQ for string length c++
1) how to find length of string in c++ without using strlen ?
As per explain in above 5 different ways, we can calculate c++ string length using string::size(), string::length(), using for loop and using while loop.
2) c++ string length vs size
Here length and size both functions of strings are exactly same, and both returns number of characters in the string which are not including any of null termination.(\0)
Both have the same complexity: Constant
size_type length() const _NOEXCEPT
{ // return length of sequence
return (this->_Mysize);
}
size_type size() const _NOEXCEPT
{ // return length of sequence
return (this->_Mysize);
}
size_type size() const noexcept;
Returns: A count of the number of char-like objects currently in the string.
size_type length() const noexcept;
Returns: size().
3) How to find array length c++
int arr[5] = {4, 1, 8, 2, 9};
int len = sizeof(arr)/sizeof(arr[0]);
array length c++ can be calculated using sizeof total array divided by first element of array.
Using Pointer also we can calculate array length c++
int arr[5] = {5, 8, 1, 3, 6};
int len = *(&arr + 1) - arr;
4) char length c++
Using same way as per string you can use strlen() function for character pointers
char* str = "char string c++";
int length = strlen(str);
cout<<length<<endl;
Conclusion:
Here we have learned different 5 methods to calculate string length in c++. We have covered all examples with and without using string functions. I hope this article will be useful for you for find string length c++. For more c++ related articles you can check our website c++ sections. Happy Coding !! ๐
Leave a Reply