C Vs. C++
As we all know, both C and C++ are significant programming languages. But do you really know what exactly makes the difference between these two languages? Ride on! Let us check it out.
Basic Knowledge about C and C++ languages
Before knowing what makes the differences between these two languages, let’s first understand the basic technicalities used in these two languages.
Let’s delve into C language:
C language is the most extensively used computer programming language. It was developed to do system programming in UNIX Operating System. C is an Imperative programming language, which was developed in the year 1969-early 1970s.
Many Operating systems like UNIX and Linux were developed using this language. Dennis Ritchie developed it at Bell laboratories. C was initially first implemented on the DEC PDP-11 computer in 1972.
Example:
#include<stdio.h>
int main()
{
printf("Hello world!");
return0;
}
Output: Hello World! |
Syntax:
“#include” is a preprocessor directive. Programmers use it to include the header files of a particular directory in our program. In the above example, we used “stdio”. It is a standard header file containing the basic functions like “printf,” “scanf,” etc.
“main()”-main() function is used in any C program because the compilation of the whole program begins from main() function.
To print a string or output on the console, we use the “printf” command. When the compiler executes this command, the string or the output statement passed as the printf function’s argument gets printed on the console window.
return 0– This statement ensures that the execution is complete, and it has reached the end of the code successfully without encountering any errors. The compiler terminates the program after the command “return”.
Facts about C language:
C language was invented and developed to build a popular operating system called UNIX. It is the successor of the “B” language.
Afterward, the UNIX operating system was developed with the help of the C language. Finally, C was formalized by the American National Standards Institute(ANSI) in the year 1988.
C is a widely used and most popular programming language. Many popular Operating systems like Linux OS and RDBMS MySQL have been written in C language.
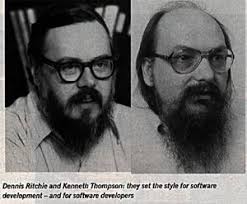
Basics about C++
C++ is a general-purpose programming language. It was an extension of C language. C++ language was developed by “Bjarne Stroustrup.” C language was developed and designed at “AT & T Bell Labs.” C++ language was developed in the year 1979.
What is striking about C is that it gives a high-level control over the system resources and memory. This is the primary reason, C is called a high-level language.
C++ is a cross-platform language that is used to create high-performance applications. C++ can be found not only in operating systems, but in GUI (Graphical User Interfaces), and in various embedded systems.
C++ is a portable language that can be adapted to multiple platforms. It is an imperative and compiled language, i.e., a compiler compiles the language into an assembly language.
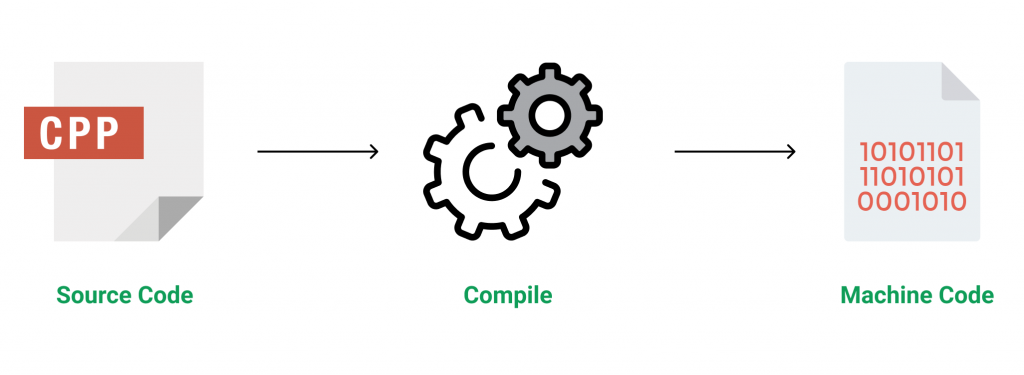
Example:
#include <iostream>
using namespace std;
int main()
{
cout<<"Hello World!";
return 0;
}
Output: Hello World! |
Syntax:
“#include” is a preprocessor directive. It is used to include the header files of a particular directory in our program. In the above example, we used “iostream”. It is a standard header file containing the basic functions like “printf”, “scanf,” etc.
“main()”- The main function consists of the body of the program. A valid C++ program must consist of this main function. Besides, only one main function can show up in a single C/ C++ source code.
“cout” command is used to display the result of the given string. Whenever this command gets executed, the statement containing inside the cout command is executed as output. Cout is similar to the printf statement in C language. They perform the same function, but share different syntax!
return 0- To specify the exit status, the return statement is used in a program. After executing the command “return,” the compiler automatically exits the program. It states the Exit status of the program.
Memory management
C++ language supports four types of memory management. By taking the help of “new” and “delete” keywords, we can easily manage the memory according to our requirements.
new– the new keyword is used to create a memory for a new variable.
Syntax: pointer_variable = new data-type;
ex: int *c = new int[2];
delete- delete keyword is used to delete the allocated memory.
Syntax: delete pointer_variable;
ex: delete c;
New and Delete are beneficial keywords when it comes to making the program less costly in terms of space. Hence, we should make it a usual practice to include these in our code.
Keywords:
Keywords are the reserved words that we cannot declare as variables in our program. Each keyword has its own meaning and unique action to perform.
auto | break | Case | char |
continue | const | do | default |
double | else | enum | extern |
for | it | goto | float |
int | long | return | register |
signed | static | sizeof | short |
struct | switch | typedef | union |
void | while | volatile | unsigned |
Facts about C++ language, YOU NEVER KNEW!
C++ language was first standardized in the year 1998. C++ has more than 35 Operators. Initially, C++ was called “The New C.” Both the languages, C and C++, were invented at AT& T bell labs.
C++ got Object-Oriented features from Simula67 language. It has 84 keywords and consists of Inline functions which are borrowed from Ada programming language.
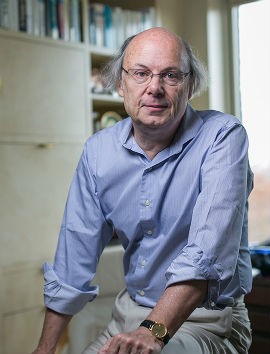
Difference between C and C++ programming (C vs C++ and C++ vs C):
Here in this section, we will discuss what makes C language different from C++.
C Language | C++ |
---|---|
C is a Procedure-Oriented Programming Language. | C++ is Object-Oriented Programming Language. |
The program is divided into small buckets of code called Functions. | The program runs on the variables of manually created data structures (classes), called Objects. |
The importance is given to Functions but not data. | The preference is mainly given to data rather than functions because data is more important in the real world. |
C language follows Top-Down approach. | C++ language follows Bottom-up approach. |
C language doesn’t have any access specifier. | C++ have access specifiers like Public, Private, Protected, etc. These are very useful when it comes to the protection of the data and providing the right access rights to different users. |
Data can move freely from function to another in C. | Data cannot move freely from one function to the other. |
C language doesn’t have any proper way of hiding data. | C++ provides Data Hiding. |
C language does not provide security to the data. | C++ language offers protection to the data. |
C language doesn’t have any namespace feature. | C++ language has a namespace feature, which is very useful. |
C language doesn’t support function overloading. | C++ supports function overloading. |
C language does not support Object overloading. | C++ language supports Object overloading. It has many useful features. |
C language does not allow functions to be defined inside the structures. | In C++, functions can be used inside a structure. A real-life application being classes! |
C language does not support reference variables. | C++ supports reference variables. |
C language has no support for virtual function. | C++ supports the virtual function. |
C language uses “scanf/printf” for input/output functions. | C++ uses “cin/cout” for input/output functions. |
Why should you use C over C++ ?
Due to the absence of an Object-Oriented Paradigm, C supports no function overloading or Operator Overloading problems. C is the most popular and acceptable programming language for Kernel Programming and Drivers development.
Static initialization is safe in C language, but not in C++. C is more suitable for low-level environments such as embedded systems because it requires less run-time support. C language is widely used in many operating systems.
C is better than C++ in speed and in efficiency too. C is better than C++ in some areas because it does not look up for VMT (Virtual Method Table).
Also, it is comparatively much more comfortable to code and debug in C rather than in C++. C is a default choice for source-level programming.
Do you know why we prefer C++ over C?
Let’s Differentiate! C++ Vs. C
Object-oriented: We all know that C++ is an Object-oriented language, making it far more useful than C language. Object orientation helps C++ to make maintainable and extensible programs.
Rich standard library support: C++ has a vast library for fast and rapid development of the applications.
Mid-level language: Since C++ is a mid-level language, we can use it for both system programming such as Kernel Programming and application development such as Media player, Game engines, etc.
C++ supports polymorphism, encapsulation, and inheritance. Both the built-in and user-defined data types are supported in C++. Hence, it supports the virtual function and Friend function also.
C++ contains 95 keywords. However, C contains only 32 keywords.
C++ supports the mechanism of overloading. i.e., Function Overloading and Operator overloading. It provides security to the data with the help of access specifiers. Moreover, C++ offers an easy way to add new data to the functions.
Now, put some light on the Pros and Cons Section.
Advantages of C
C language has Dynamic memory allocation. We can add or delete the memory of a particular variable through Dynamic memory allocation.
C language is portable; i.e., it can be used irrespective of the system. It has various built-in functions like puts(), gets(), etc. These functions reduce the manual process of rewriting the code to get done the desired work.
C is a middle-level language. The advantage of middle-level language is that we can do Kernel Programming and gaming engines, etc.
C language is user friendly, which makes it easier to learn.
Disadvantages of C
C language does not have any object-oriented features like C++. No security is provided to the data. It lacks the namespace facility. C language does not support polymorphism, encapsulation, or inheritance.
C language lacks the feature of overloading. i.e., Function and Method Overloading. It neither has constructors nor Destructors. Hence, it lacks the Run-time checking feature.
Advantages of C++ over C:
C++ is an Object-oriented programming language. Unlike C language, it has all the features of Polymorphism, Inheritance, Classes, etc. C++ compiler also compiles everything that is done by the C compiler, which makes it more robust.
C++ also provides us with a Data hiding facility that is not available is C.
C++ also supports the virtual function and friend function. It also supports the reference variables which lacks in C language.
Overloading:
We can easily overload the functions in C++. Function overloading and method overloading is the most prominent feature which lacks in C and is present in C++
Disadvantages:
C++ does not support the Garbage collection feature. It also does not provide support for the threads built-in.
C++ is a complex language. When compared to the C language, the C++ compiler is relatively slow. C++ has no security because it has pointers, friend functions, and also global variables.
Now, you just need to check your or the client’s requirements in terms of security and other basic features and then decide which out of C or C++ will be the best language for the making of the application.
Do you still have a doubt? No problem! Let’s clear that too!
Are C and C++ both the same?
The answer is a big NO. Even though they share a standard syntax, C is a Procedure oriented programming language whereas C++ is an Object-oriented programming language. One cannot perform overloading and inheritance in C language. On a broad scale, they may look the same, but they are totally different.
Conclusion
With this question, let us end the discrimination between C++ and C !
In this article, we started with imparting some basic knowledge about these two significant programming languages. Then we put light on some of the most basic and essential features. Then we shifted to some advantages and disadvantages of these languages.
We can never compare two languages without considering some real-life scenarios. Hence, in the end, we looked at some of the desired features in real-life applications. Which features are provided by which language out of the two? We saw all this detail.
Hence, look at your requirements, and now you know which language is best suited for your application now.
I hope this article helped.
All the best and Keep Coding!
Leave a Reply