During writing logic in C++, many times we come across where we require to convert character to an Integer value. We have collected different methods that used to convert char to int C++.
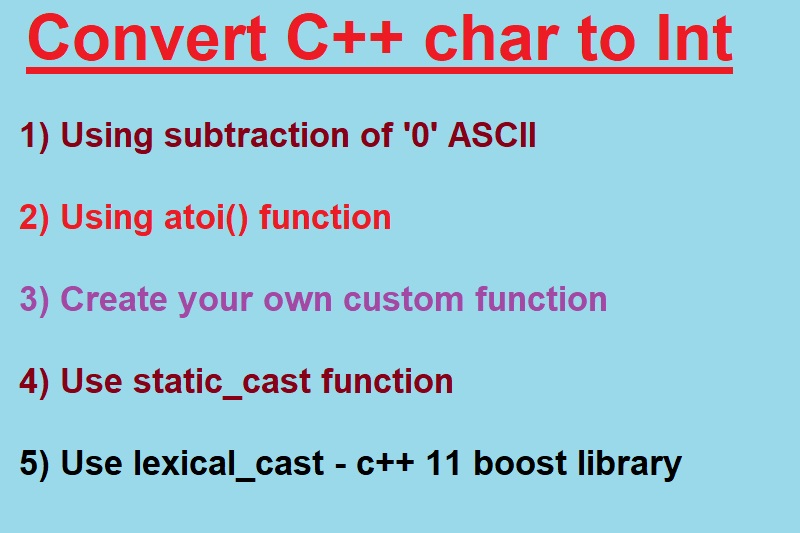
1) Convert C++ char to Int using subtraction of ‘0’ ASCII value.
char chDigit = '5';
// By substracting character
ConvertedValue = chDigit - '0';
cout << "1) Using substract '0' : "<< ConvertedValue<<endl;
// By substracting ASCII value
ConvertedValue = chDigit - 48;
cout << "2) By substract ASCII of '0' : " << ConvertedValue<<endl;
Input : ‘5’ , Output: 5
Here we are subtracting ‘0’ from character. So char to Int ascii conversion will happens. Same code can be use for char to int c programming also.
‘5’ – ‘0’ means 53 – 48 = 5 . So given method will be used for single digits. This is the first way for c++ convert char to int.
2) C++ convert char to Int using atoi() function
There is C library function atoi() is available. It is inside stdlib.h file.
const char* chpMultiDigit = "123";
ConvertedValue = atoi(chpMultiDigit);
cout << "3) Using atoi : " << ConvertedValue << endl;
Input : ‘123’ , Output: 123
Here we have used the character pointer variable which is used to assign string value. Atoi() function will convert string to integer c++.
This is general way use in C programming also for c++ char to int.
3) Convert char to Int C++ using custom function code
int CustomAtoi(const char* str)
{
// Initialize result
int Result = 0;
// Iterate through all characters
for (int Index = 0; str[Index]!= '\0'; Index++)
Result = Result * 10 + str[Index] - '0';
// return result.
return Result;
}
Here CustomAtoi function will convert string to integer using custom logic. Many time in interview of c++ you will get question like How to convert string to integer or char to int without using atoi() function ? Then you have to create your own custom function for it.
If we know exact value digit then we can write simple code also.
const char* chpMultiDigit = "123";
ConvertedValue = (chpMultiDigit[0] - '0') * 100 + (chpMultiDigit[1] - '0') * 10 + (chpMultiDigit[2] - '0');
cout << "4) Using char : " << ConvertedValue << endl;
4) c++ convert char to Int using static_cast function
In C++ casting functions are also available. static_cast is used to convert data from one data type to another. example: float to Int. or char to Int. But here it will not covert direct char to int value. it will convert and give ASCII value of character.
char chDigit = '5';
ConvertedValue = static_cast<int>(chDigit);
cout << "6) Using static_cast: " << ConvertedValue << endl;
Input: ‘5’ , Output: 53
same way you can direct cast value to int in C++.
char chAlpha = 'a';
ConvertedValue = (int)(chAlpha);
cout << "7) Using typecast: " << ConvertedValue << endl;
Input: ‘a’ , Output: 97
char to int ascii table used for C and C++
If you are not aware of all ASCII value. Please check below table.
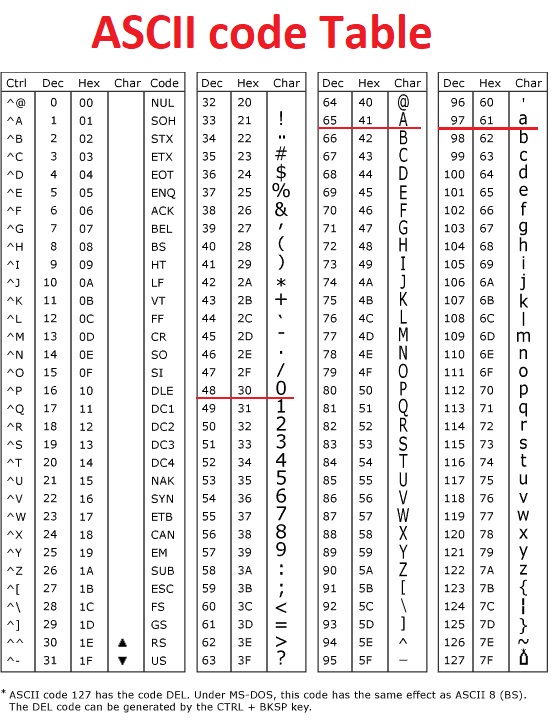
5) Convert char to Int c++ using boost library lexical cast
In C++11 and Boost library there is special casting function is available. It is C++ Template based casting function. For using this function you will require to use Boost library in you project. You can download and configure boost library and then you will have access to many features of boost library. It contain lot of algorithms also.
#include "boost/lexical_cast.hpp"
ConvertedValue = lexical_cast<int>(chDigit);
Here is full c++ char to int conversion code.
#include<iostream>
using namespace std;
int CustomAtoi(const char* str)
{
// Initialize result
int Result = 0;
// Iterate through all characters
for (int Index = 0; str[Index]!= '\0'; Index++)
Result = Result * 10 + str[Index] - '0';
// return result.
return Result;
}
int main()
{
char chDigit = '5';
const char* chpMultiDigit = "123";
char chAlpha = 'a';
int ConvertedValue;
ConvertedValue = chDigit - '0';
cout << "1) Using substract '0' : "<< ConvertedValue<<endl;
ConvertedValue = chDigit - 48;
cout << "2) By substract ASCII of '0' : " << ConvertedValue<<endl;
ConvertedValue = atoi(chpMultiDigit);
cout << "3) Using atoi : " << ConvertedValue << endl;
ConvertedValue = (chpMultiDigit[0] - '0') * 100 + (chpMultiDigit[1] - '0') * 10
+ (chpMultiDigit[2] - '0');
cout << "4) Using char : " << ConvertedValue << endl;
ConvertedValue = CustomAtoi(chpMultiDigit);
cout << "5) Using CustomAtoi : " << ConvertedValue << endl;
ConvertedValue = static_cast<int>(chDigit);
cout << "6) Using static_cast: " << ConvertedValue << endl;
ConvertedValue = (int)(chAlpha);
cout << "7) Using typecast: " << ConvertedValue << endl;
/*
// Used in C++11 / Boost library
#include "boost/lexical_cast.hpp"
ConvertedValue = lexical_cast<int>(chDigit);
*/
return 0;
}
After executing this code you will get below output:
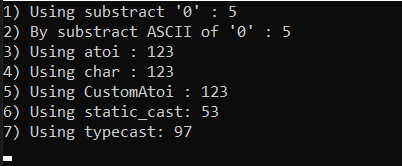
Output:
1) Using substract ‘0’ : 5
2) By substract ASCII of ‘0’ : 5
3) Using atoi : 123
4) Using char : 123
5) Using CustomAtoi : 123
6) Using static_cast: 53
7) Using typecast: 97
Conclusion of char to int conversion:
I hope you will get your problem resolution from given different methods of converting char to int c++. So mainly you can use subtracting ‘0’ or its ASCII value or if there is the string to int require you can directly use atoi() function. In case your need is not satisfied you need to create your custom function for c++ char to int conversion.
In case you did not found your solution please comment below, we will try to help you and resolve your issue.
Till now happy coding !!
Leave a Reply