Vector in c++ is like a dynamic array, but it has the ability to resize whenever we are adding or deleting elements from vector. Vector is a type of Sequence container. Containers hold data of the same data types. Apart from sequence containers, there are different types of containers available in vector.
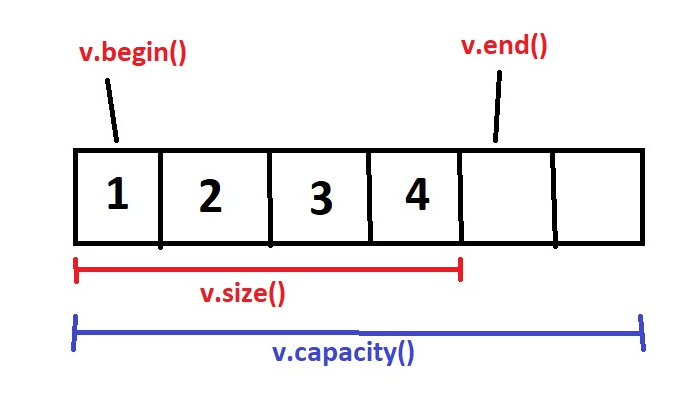
If we check internally, vectors are also dynamically allocated array which store elements. If more elements increase inside vector then it automatically increases the size of dynamically allocated array. Internally it increases its capacity. Expanding memory allocation every time is an expensive task, that’s why vector allocates a few capacities every time rather than single memory block allocation.
Sequence containers are vector, list, dequeue, arrays, and forward_list
Container Adaptors are Queue, priority_queue, stack
Associative containers are set, multiset, map, and multimap
Unordered associative containers are unordered_set, unordered_multiset, unordered_map and unordered_multimap
Elements of vectors are stored in continues memory location, so it is easy to print vector c++.
Print vector in C++
#include<iostream>
#include<vector>
using namespace std;
int main()
{
vector<int> vData;
vData.push_back(1);
vData.push_back(2);
vData.push_back(3);
vData.push_back(4);
vector<int>::iterator it;
for (it = vData.begin(); it != vData.end(); it++)
{
cout << *it <<endl;
}
return 0;
}
Output:
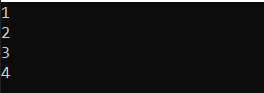
For using vector you need to include #include<vector> in header. For creating vector in c++ you have to use vector<datatype> objectName;
push_back(value) is used in vector for adding new value inside vector. push_back function will always add value at the end of vector. In case you want to add element in front of vector then there is push_front() function is also available.
How to print vector in c++ ?
For printing vector in c++ you have to create iterator which will iterate throughout the vector and print element one by one. you can create iterator by given syntax.
vector<DataType>::Iterator ObjectName;
Now you can use iterator object in for loop for iterating first element to last element. We can assign starting as vector begin() function and end as vector end() function. So we will continue for loop untill iterator is not end().
For printing value of vector in c++ we use pointer of iterator object. *it will give value of vector which we can easily print.
Print vector in c++ using auto keyword (c++ 11 feature)
for (auto it = vData.begin(); it != vData.end(); it++)
{
cout << *it << endl;
}
It will also give similar output and print vector in c++. Using auto keyword we will not require to declare iterator because auto will automatically identify which type require to hold value. So here it will automatically identify that it will require vector<int>::iterator datatype. Given auto keyword feature is introduced in c++ 11.
C++ print vector in reverse order
After looking above example you must have question someone want c++ print vector in reverse order then ? Yes, STL (Standard Template Library) is providing reverse traversal by rbegin() and rend() functions.
for (auto it = vData.rbegin(); it != vData.rend(); it++)
{
cout << *it << endl;
}
Output :
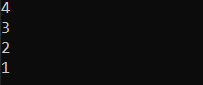
Conclusion:
If you want to print vector in C++ then you can use iterator and traverse loop and print via pointer of iterator object. If your project/IDE is supporting c++ 11 then i will recommend you to use auto keyword for traversing for loop and display value.
Many thanks for discussing this fantastic written content on your website. I came across it on google. I may check to come back once you post additional aricles.