In c++ incomplete type is not allowed error occurs when compiler detect any identifier that is of known data type but definition of it’s is not seen fully.
Below are general errors in c++:
- incomplete type is not allowed
- stringstream incomplete type is not allowed
- ifstream incomplete type is not allowed
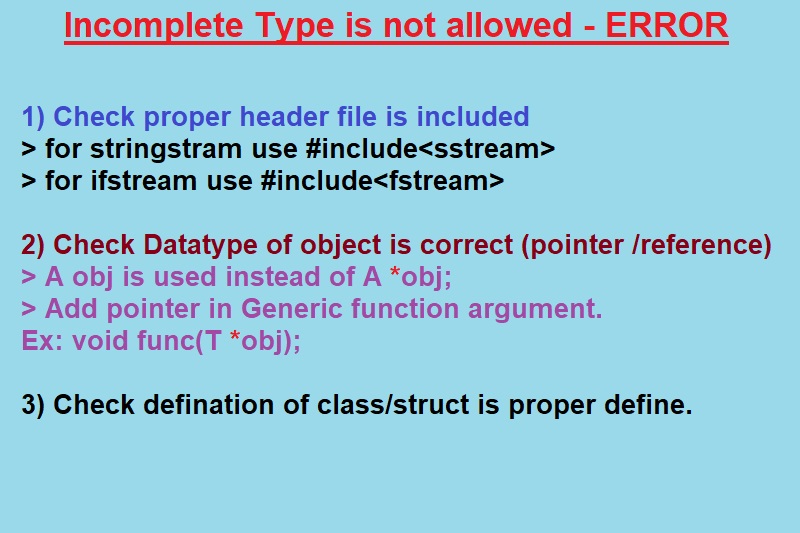
Always check below point for these type of error:
- Have you included proper header file require to use?
- Check data type of object is correct ? Many times it require pointer(*) or referee (&) but we are using simple object.
- Check definition of class/struct is available ? Possibly have done forward declaration of struct or class but it is not define properly.
c++ incomplete type is not allowed
PROBLEM-1:
#include<iostream>
class A {
public:
A Parent; //Error: Incomplete type is not allowed
A(A *ptr) : Parent(*ptr) {
}
};
SOLUTION-1:
Here object of class can not contain instance of it’s own (A Parent). Because in constructor also we have used it. And when any object create constructor will be called. So Compiler will try recursive call and it will become infinite.
For resolving this error we need to use pointer(*).
#include<iostream>
class A {
public:
A *Parent; // Pointer of class
A(A *ptr) : Parent(ptr) {
}
};
————————————
PROBLEM-2:
template<class T>
class A
{
public:
void func(T tObj);
};
void A<class T>::func(T tObj) // ERROR occurs here
{
// Do something here
}
SOLUTION-2:
template<class T>
class A
{
public:
void func(T *tObj); // Solution: Add Pointer
};
void A<class T>::func(T *tObj) // Solution: Add Pointer
{
// Do something here
}
c++ incomplete type is not allowed stringstream
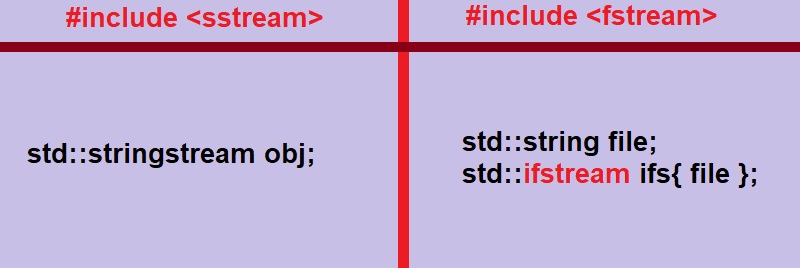
PROBLEM:
#include<iostream>
// Require to add header file
int main() {
std::stringstream obj; // ERROR: incomplete type is not allowed
return 0;
}
SOLUTION:
Here we have not included sstream header file, so compiler is not able to find std::stringstream and giving incomplete type is not allowed stringstream error. If you declare include file given error will be resolved.
#include<iostream>
#include <sstream> // Require to use std::stringstream
int main() {
std::stringstream obj; //RESOLVED by adding header
return 0;
}
ifstream incomplete type is not allowed
PROBLEM:
#include <iostream>
// require header file
int main()
{
std::string file;
std::ifstream ifs{ file }; //ERROR : incomplete type is not allowed
}
SOLUTION:
In c++ for using ifstream, we require #include <fstream> otherwise compiler will not able for find it properly and will give you error of ifstream incomplete type is not allowed.
#include <iostream>
#include <fstream> // Require for using ifstream
int main()
{
std::string file;
std::ifstream ifs{ file }; //RESOLVED by adding header
}
SEE MORE:
Leave a Reply